Multipliers
Array Multiplier
- Shift and add principle
- For x,y≥0
- xy=(∑i=0n−1xi2i)(∑j=0n−1yj2j)=∑i=0n−1(2i⋅∑xiyj2j)
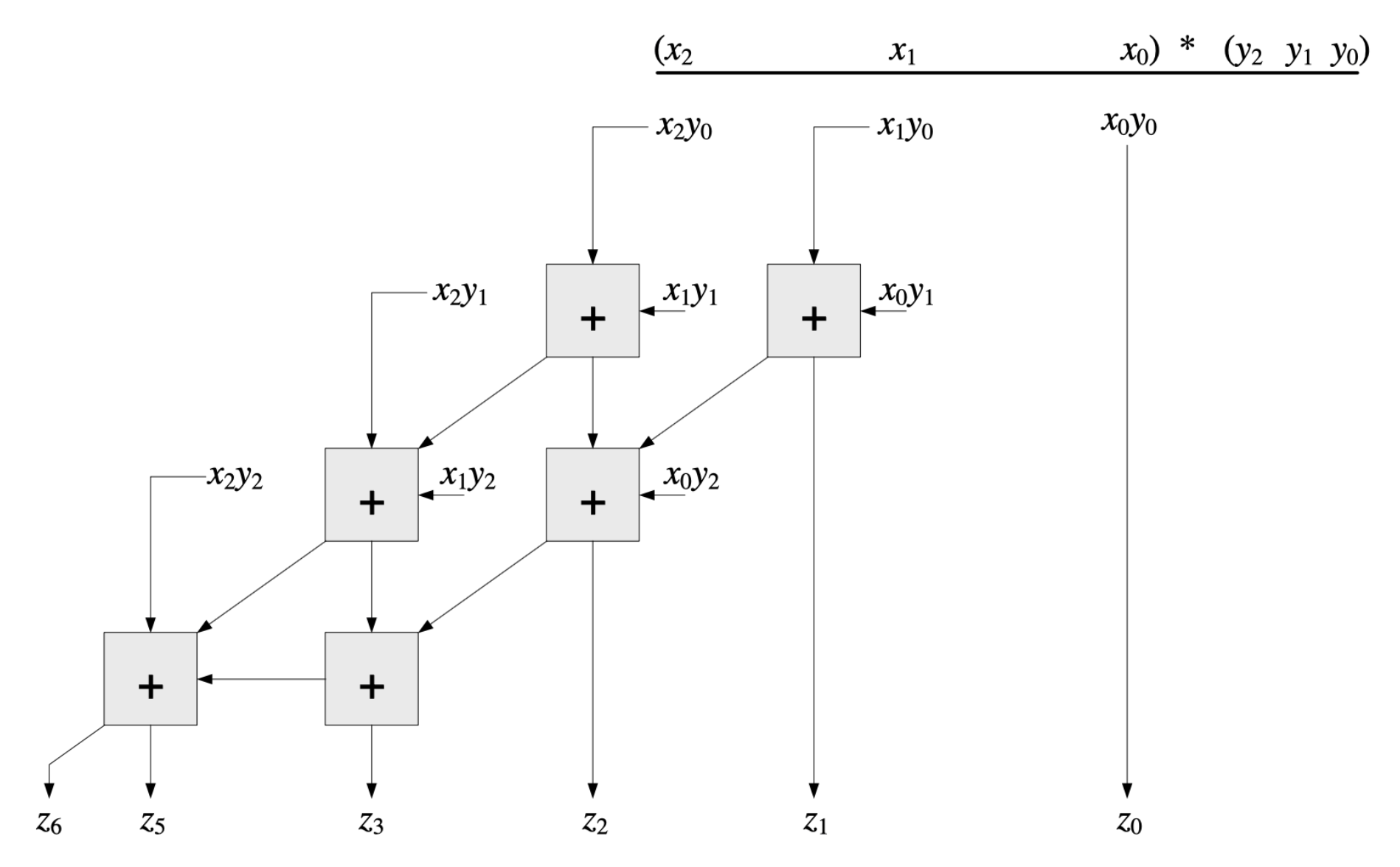
- Overhead
- Hardware: O(n2) gates
- n2 2-bit multiplications: n2 2-AND gates
- n n-bit additions: n(n−1) full adders
- Delay: O(n)
Sequential Multiplier
- Partial sums are accumulated stepwise
- Notes
- Y is stored in a partial sum register → save 1 register
- ‘logic shift’, i.e. fill right with 0s
- Algorithm
- Load X,Y
- C←0, P←0
- Iterate n-times:
- if Y(0)=1
- then P←P+X
- else P←P+0
- shift (C,P,Y) 1 digit left
- Result is in (P,Y)
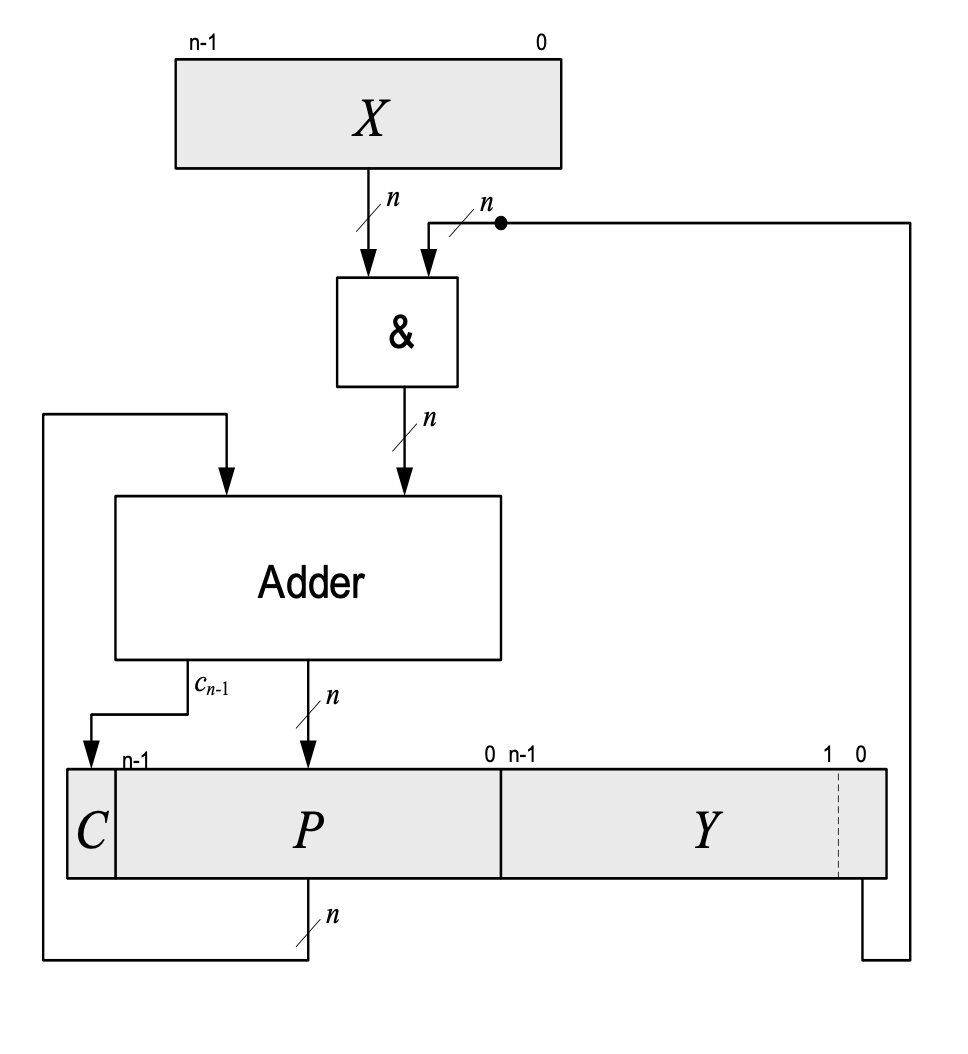
Signs
- Sign Magnitude
- Compute magnitude and sign x=(sx∣x∣),y=(sy∣y∣)
- Multiply the magnitudes
- Compute the sign of the result sz=sx⊕sy
- Negate the result if negative
- 2’s Complement
- If x<0
- ‘Arithmetic shift’ (signed shift, sign extension) : shift left with sign instead of 0
- Sign in position pn−1; on overflow in cn−1 → shift pn−1+cn−1
- If y<0
- Then (2n−∣y∣)x is what was computed
- We need to subtract 2nx through P←P–x to get the correct result
Booth Multiplier
- Optimization
- No need to add when yi=0, only shift
- It is not necessary to perform (m+1) additions when there is a chain of (m+1) 1‘s in y
- We just need to subtract X at the begin of the chain and add X back at the end of the chain
- x∑j=ii+m2i=x(∑j=0i+m2j−∑l=0i−12l)=x(2i+m+l−1−2i+1)=x(2i+m+l−2i)
- Where i is the first digit that is 1 and m is the number of consecutive 1s (so i+m is the last 1 in the chain)
- Booth Recoding: y is translated to y′
- y is extended by y−1=0
- y′ is built as follows yi′=yi−1–yi
- Meaning of y′:
- y′=0: shift right
- y′=−1 (represented “S”): P –= x then right shift (begin of a chain of 1s)
- y′=1 (represented “A”) : P += X then right shift (end of a chain of 1s)
- Requires an addition and subtraction for each chain
- Weakness: when multiplier alternates between 0s and 1s
- Can sometimes be avoided by switching the first and second operands